Overleaf
Reimplementation of diagrams from https://www.overleaf.com/learn/latex/Feynman_diagrams
[2]:
from pyfeyn2.feynmandiagram import FeynmanDiagram, Leg, Propagator, Vertex
from pyfeyn2.render.all import AllRender
from pyfeyn2.auto.position import feynman_adjust_points
[3]:
v1 = Vertex().with_xy(-1, 0)
v2 = Vertex().with_xy(1, 0)
fd = FeynmanDiagram().add(
v1,
v2,
Propagator().connect(v1, v2).with_type("photon").put_style('opacity',0.2).put_style('color','red').with_momentum("$k$"),
Leg().with_target(v1).with_xy(-2, 1).with_type("fermion").with_incoming().with_label("$e^+$").put_style('opacity',0.2),
Leg().with_target(v1).with_xy(-2, -1).with_type("fermion").with_incoming().with_label("$e^-$").put_style('opacity',0.2),
Leg().with_target(v2).with_xy(2, 1).with_type("fermion").with_outgoing().with_label("$\\mu^+$").put_style('opacity',0.2),
Leg().with_target(v2).with_xy(2, -1).with_type("fermion").with_outgoing().with_label("$\\mu^-$").put_style('opacity',0.9),
)
ar = AllRender(fd)
ar.render()
pyx:
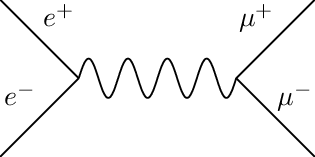
feynmp:
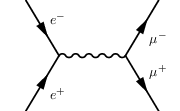
tikz:
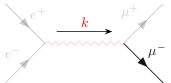
dot:
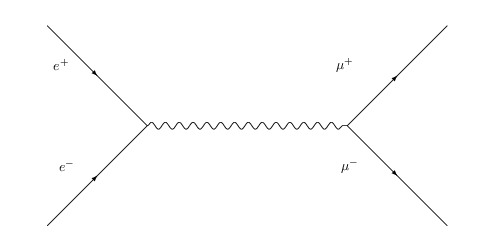
feynman:
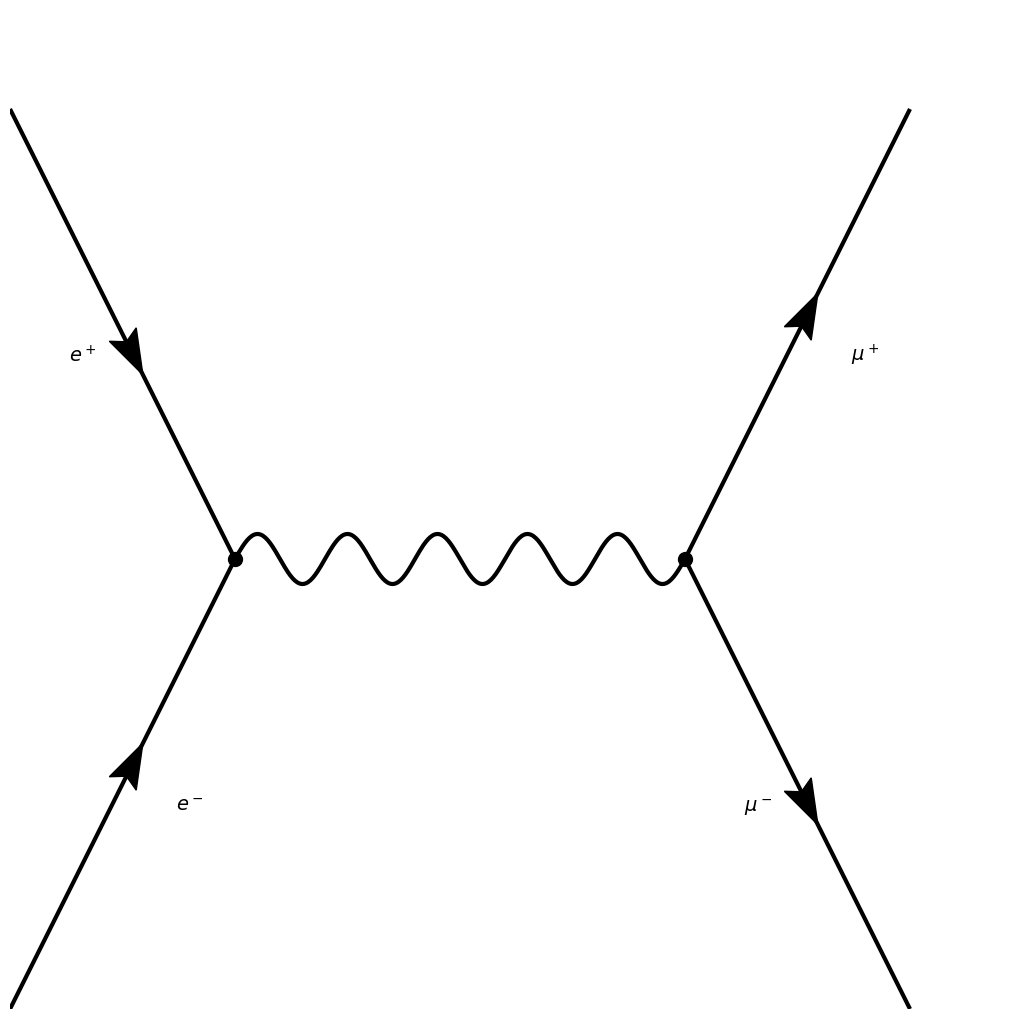
mpl:
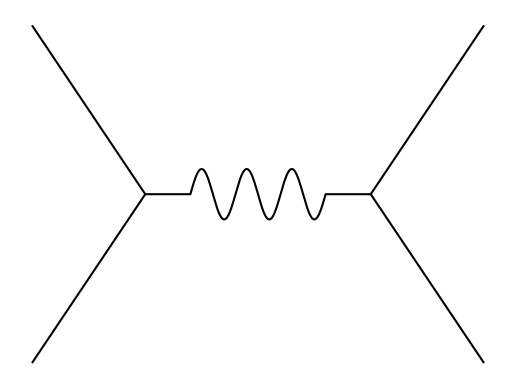
ascii:
* *
-> >--
-- e+ -
-- + ---
>- >
*~~~~~~~~~~~~~~~~~~~*-
>- >
-- ----
e- -- -
-> >--
* *
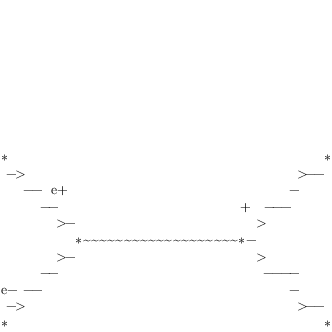
unicode:
* *
↘↘ ↗↗↗
↘↘ e^ + ↗
↘↘ + μ^ ↗↗↗
↘↘ ↗
*~~~~~~~~~~~~~~~~~~~*↘
↗↗ ↘
- ↗↗ ↘↘↘μ^
e^ ↗↗ ↘ -
↗↗ ↘↘↘
* *
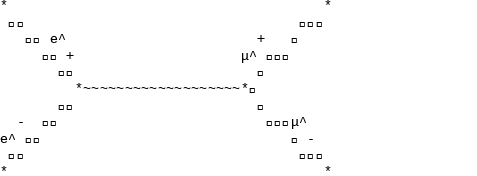
[4]:
v1 = Vertex().with_xy(-1, 0)
v2 = Vertex().with_xy(1, 0)
fd = FeynmanDiagram().add(
v1,
v2,
Propagator().connect(v1, v2).with_type("fermion"),
Leg().with_target(v1).with_xy(-2, 1).with_type("fermion").with_incoming(),
Leg().with_target(v1).with_xy(-2, -1).with_type("photon").with_incoming(),
Leg().with_target(v2).with_xy(2, 1).with_type("fermion").with_outgoing(),
Leg().with_target(v2).with_xy(2, -1).with_type("photon").with_outgoing(),
)
ar = AllRender(fd)
ar.render()
pyx:
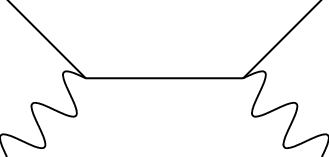
feynmp:
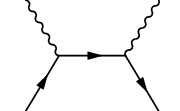
tikz:
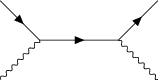
dot:
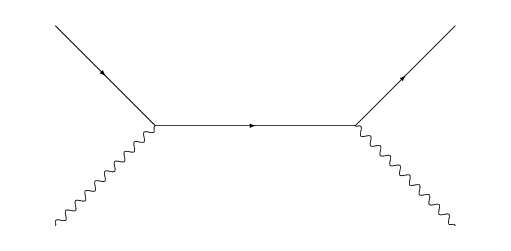
feynman:
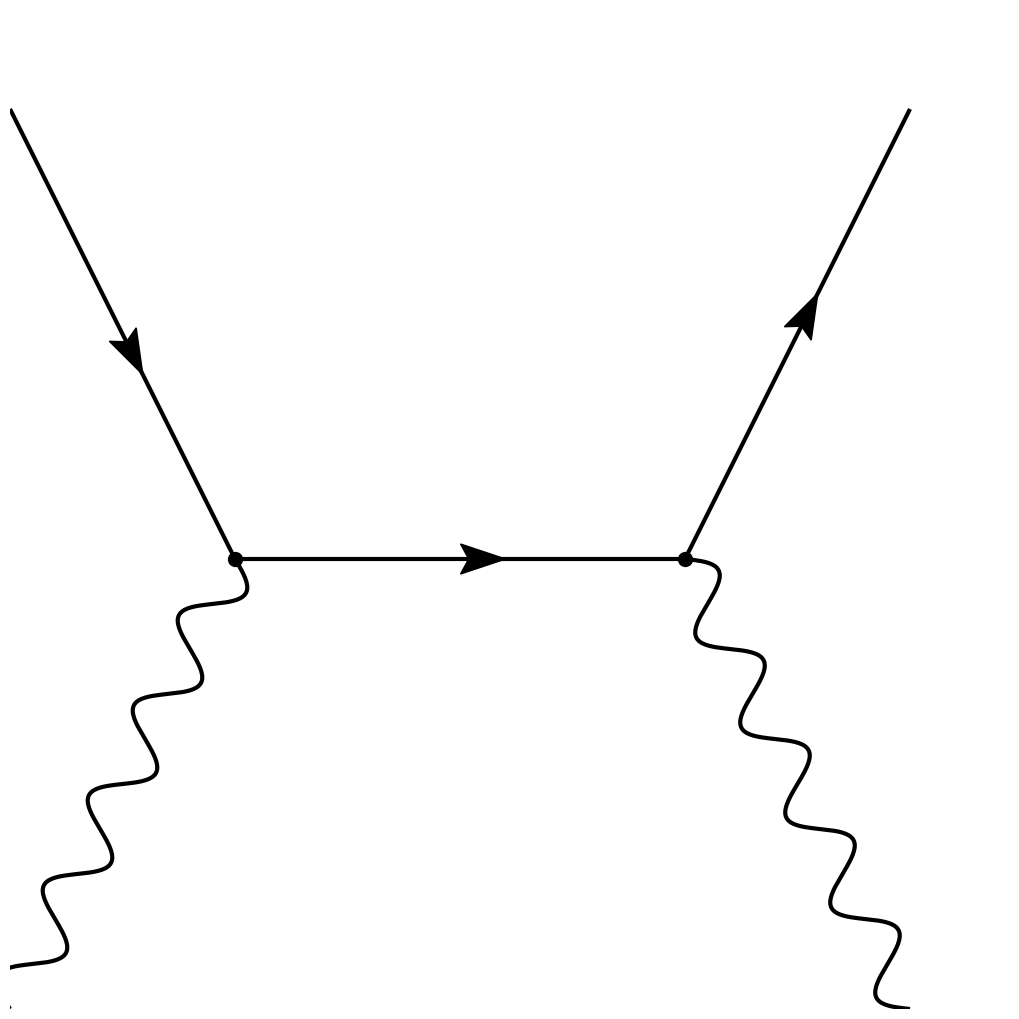
mpl:
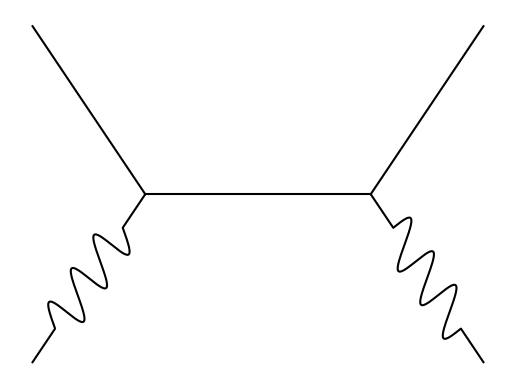
ascii:
* *
-> >--
-- -
-- ---
>- >
*->---->---->---->--*~
~~ ~
~~ ~~~
~~ ~
~~ ~~~
* *
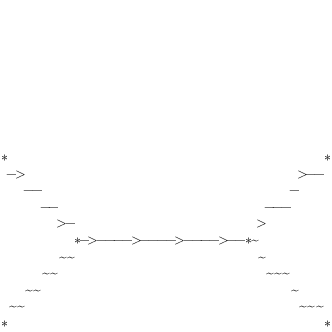
unicode:
* *
↘↘ ↗↗↗
↘↘ ↗
↘↘ ↗↗↗
↘↘ ↗
*→→→→→→→→→→→→→→→→→→→*~
~~ ~
~~ ~~~
~~ ~
~~ ~~~
* *
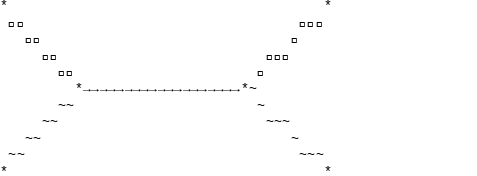
[5]:
v1 = Vertex().with_xy(-2, -2)
v2 = Vertex().with_xy(2, -2)
i1 = Vertex().with_xy(-2, 2)
o1 = Vertex().with_xy(2, 2)
o2 = Vertex().with_xy(2, 1)
o3 = Vertex().with_xy(2, 0)
p1 = Vertex().with_xy(0, 2)
p2 = Vertex().with_xy(1, 1)
fd = FeynmanDiagram().add(
v1,
v2,
Propagator().connect(v1,v2).with_type("fermion").with_label("$d$").with_tension(0),
Leg().with_target(v1).with_point(v1).with_type("phantom").with_incoming(),
Leg().with_target(v2).with_point(v2).with_type("phantom").with_outgoing(),
i1,p1,p2,o1,o2,o3,
Propagator().connect(p2,p1).with_type("boson").with_label("$W^+$"),
Leg(label=r"$\bar{b}$").with_target(p1).with_point(i1).with_type("fermion").with_incoming(),
Leg(label=r"$\bar{c}$").with_target(p1).with_point(o1).with_type("fermion").with_outgoing(),
Leg(label="$c$").with_target(p2).with_point(o2).with_type("fermion").with_outgoing(),
Leg(label=r"$\bar{s}$").with_target(p2).with_point(o3).with_type("fermion").with_outgoing()
)
ar = AllRender(fd)
ar.render()
pyx:
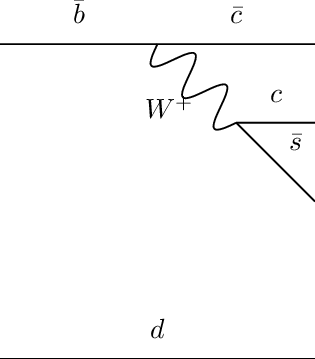
feynmp:
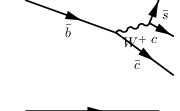
tikz:
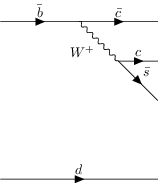
dot:
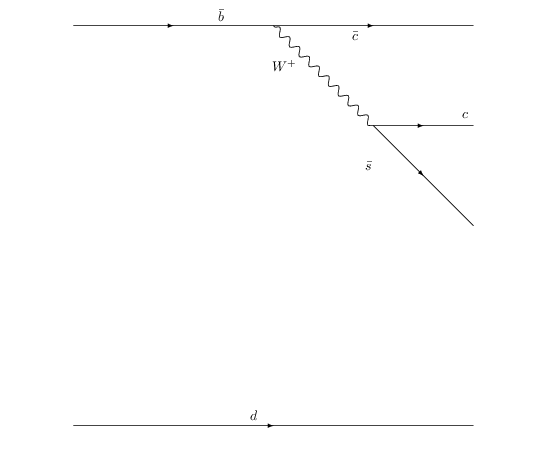
feynman:
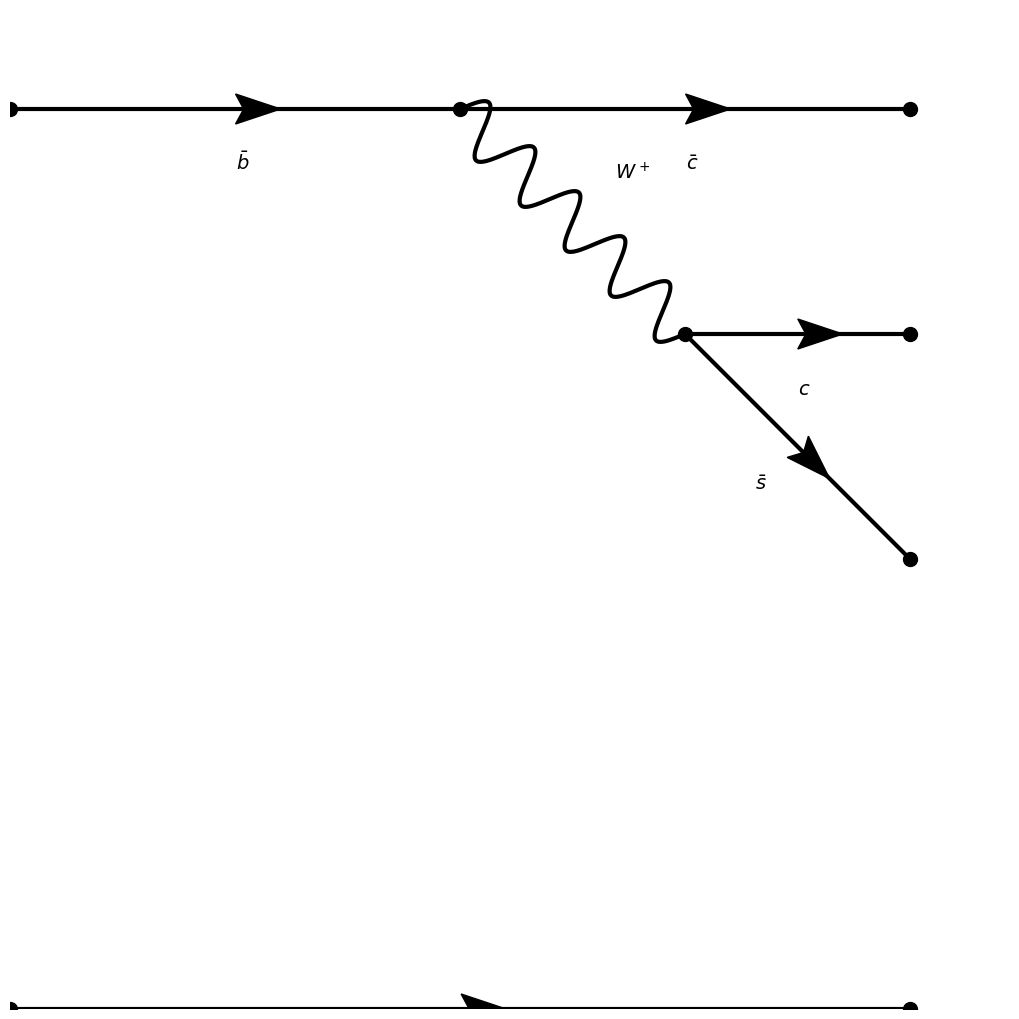
mpl:
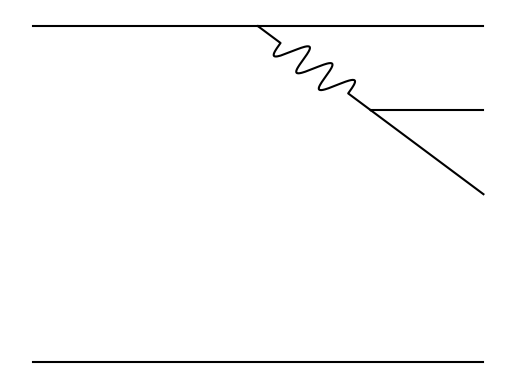
ascii:
_b _c
*->---->---->---->-*->---->---->---->--*
~~~
~ c
+ ~~~
W ~
~*->---->--*
>
---_s
-
>--
*
d
*->---->---->---->---->---->---->---->-*
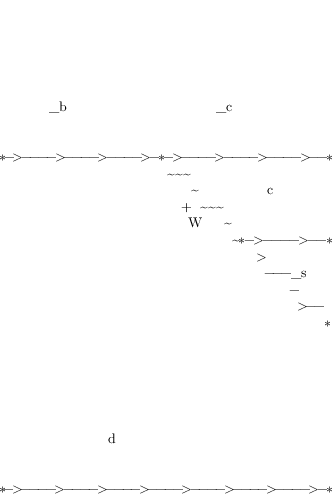
unicode:
b̅ c̅
*→→→→→→→→→→→→→→→→→→*→→→→→→→→→→→→→→→→→→→*
~~~
~ c
+^ ~~~
W ~
~*↘→→→→→→→→*
↘
↘↘↘s̅
↘
↘↘↘
*
d
*→→→→→→→→→→→→→→→→→→→→→→→→→→→→→→→→→→→→→→*
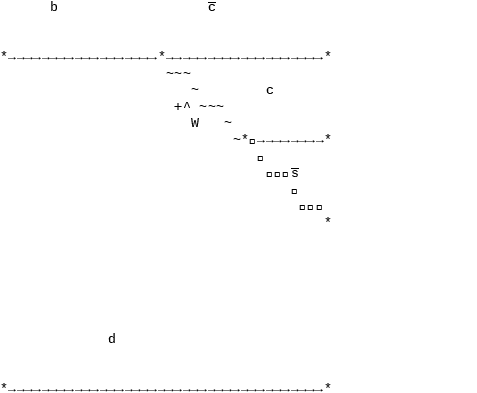
[ ]:
[ ]:
[ ]: