Dot
DotRender uses dot2tex which in turn TikZ in Latex.
https://dot2tex.readthedocs.io/en/latest/
https://github.com/kjellmf/dot2tex
https://graphviz.org/doc/info/lang.html
[1]:
from pyfeyn2.feynmandiagram import FeynmanDiagram, Leg, Propagator, Vertex
from pyfeyn2.render.latex.tikzfeynman import TikzFeynmanRender
from pyfeyn2.render.latex.dot import DotRender, feynman_to_dot
import pyfeyn2
import copy
print(pyfeyn2.__version__)
2.1.0
[2]:
fd = FeynmanDiagram()
v1 = Vertex("v1")
v2 = Vertex("v2")
v3 = Vertex("v3")
v4 = Vertex("v4")
p1 = Propagator("p1").connect(v2, v1).with_type("fermion").with_label("$\mu$")
p2 = Propagator("p2").connect(v1, v3).with_type("fermion")
p3 = Propagator("p3").connect(v3, v2).with_type("fermion")
p4 = Propagator("p4").connect(v4, v3).with_type("gluon")
p5 = Propagator("p5").connect(v4, v2).with_type("gluon")
l1 = Leg("l1").with_target(v1).with_type("gluon").with_incoming().with_xy(-2, 1)
l2 = Leg("l2").with_target(v1).with_type("gluon").with_incoming().with_xy(-2, -1)
l3 = Leg("l3").with_target(v2).with_type("gluon").with_outgoing().with_xy(2, -2)
l4 = Leg("l4").with_target(v3).with_type("gluon").with_outgoing().with_xy(2, 2)
l5 = Leg("l5").with_target(v4).with_type("gluon").with_outgoing().with_xy(2, 1)
l6 = Leg("l6").with_target(v4).with_type("gluon").with_outgoing().with_xy(2, -1)
fd.propagators.extend([p1, p2, p3, p4, p5])
fd.vertices.extend([v1, v2, v3, v4])
fd.legs.extend([l1, l2, l3, l4, l5, l6])
[3]:
dr= DotRender(fd)
[4]:
print(dr.get_src_dot())
graph G {
rankdir=RL;
layout=neato;
node [style="invis"];
l1 [ pos="-2.0,1.0!"];
l2 [ pos="-2.0,-1.0!"];
l3 [ pos="2.0,-2.0!"];
l4 [ pos="2.0,2.0!"];
l5 [ pos="2.0,1.0!"];
l6 [ pos="2.0,-1.0!"];
edge [style="decorate,postaction={decorate,draw,red,decoration={markings,mark=at position 0.5 with {\arrow{>}}}}",texmode="raw",label="$\mu$"];
v2 -- v1;
edge [style="decorate,postaction={decorate,draw,red,decoration={markings,mark=at position 0.5 with {\arrow{>}}}}",texmode="raw",label=""];
v1 -- v3;
edge [style="decorate,postaction={decorate,draw,red,decoration={markings,mark=at position 0.5 with {\arrow{>}}}}",texmode="raw",label=""];
v3 -- v2;
edge [style="decorate,decoration={coil,aspect=0.3,segment length=1mm}",texmode="raw",label=""];
v4 -- v3;
edge [style="decorate,decoration={coil,aspect=0.3,segment length=1mm}",texmode="raw",label=""];
v4 -- v2;
edge [style="decorate,decoration={coil,aspect=0.3,segment length=1mm}",texmode="raw",label=""];
l1 -- v1;
edge [style="decorate,decoration={coil,aspect=0.3,segment length=1mm}",texmode="raw",label=""];
l2 -- v1;
edge [style="decorate,decoration={coil,aspect=0.3,segment length=1mm}",texmode="raw",label=""];
v2 -- l3;
edge [style="decorate,decoration={coil,aspect=0.3,segment length=1mm}",texmode="raw",label=""];
v3 -- l4;
edge [style="decorate,decoration={coil,aspect=0.3,segment length=1mm}",texmode="raw",label=""];
v4 -- l5;
edge [style="decorate,decoration={coil,aspect=0.3,segment length=1mm}",texmode="raw",label=""];
v4 -- l6;
{rank=min; l1 l2 }
{rank=max; l3 ;l4 ;l5 ;l6 ;}
}
Render Dot script via dot2tex, so tikz again. No need to fix points via dot here explicitly.
[5]:
dr.render(resolution=100)
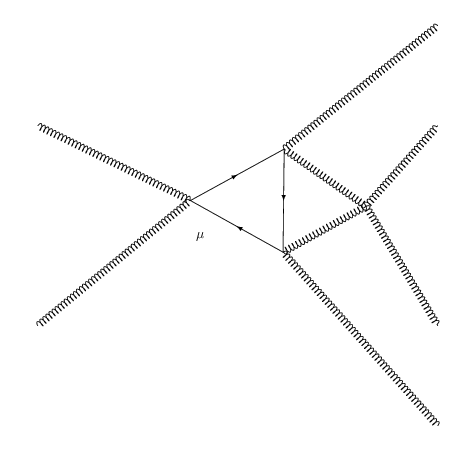
[5]:
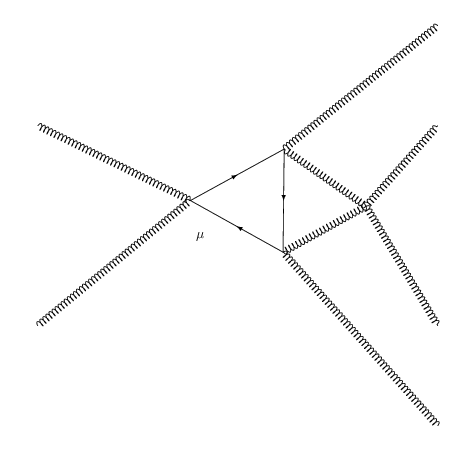
[6]:
print(dr.get_src_diag())
\begin{tikzpicture}[>=latex,line join=bevel,]
%%
\begin{scope}
\pgfsetstrokecolor{black}
\definecolor{strokecol}{rgb}{1.0,1.0,1.0};
\pgfsetstrokecolor{strokecol}
\definecolor{fillcol}{rgb}{1.0,1.0,1.0};
\pgfsetfillcolor{fillcol}
\filldraw (0.0bp,0.0bp) -- (0.0bp,324.0bp) -- (342.0bp,324.0bp) -- (342.0bp,0.0bp) -- cycle;
\end{scope}
\begin{scope}
\pgfsetstrokecolor{black}
\definecolor{strokecol}{rgb}{1.0,1.0,1.0};
\pgfsetstrokecolor{strokecol}
\definecolor{fillcol}{rgb}{1.0,1.0,1.0};
\pgfsetfillcolor{fillcol}
\filldraw (0.0bp,0.0bp) -- (0.0bp,324.0bp) -- (342.0bp,324.0bp) -- (342.0bp,0.0bp) -- cycle;
\end{scope}
\coordinate (l1) at (27.0bp,234.0bp);
\coordinate (l2) at (27.0bp,90.0bp);
\coordinate (l3) at (315.0bp,18.0bp);
\coordinate (l4) at (315.0bp,306.0bp);
\coordinate (l5) at (315.0bp,234.0bp);
\coordinate (l6) at (315.0bp,90.0bp);
\coordinate (v1) at (136.9bp,180.13bp);
\coordinate (v2) at (203.59bp,142.95bp);
\coordinate (v3) at (204.41bp,217.19bp);
\coordinate (v4) at (263.32bp,176.05bp);
\draw [decorate,decoration={coil,aspect=0.3,segment length=1mm}] (l1) ..controls (68.145bp,213.83bp) and (95.809bp,200.27bp) .. (v1);
\draw [decorate,decoration={coil,aspect=0.3,segment length=1mm}] (l2) ..controls (64.82bp,121.01bp) and (99.259bp,149.26bp) .. (v1);
\draw [decorate,decoration={coil,aspect=0.3,segment length=1mm}] (v2) ..controls (238.83bp,103.43bp) and (279.49bp,57.83bp) .. (l3);
\draw [decorate,postaction={decorate,draw,red,decoration={markings,mark=at position 0.5 with {\arrow{>}}}}] (v2) ..controls (174.91bp,158.94bp) and (165.83bp,164.0bp) .. (v1);
\definecolor{strokecol}{rgb}{0.0,0.0,0.0};
\pgfsetstrokecolor{strokecol}
\draw (143.86bp,153.98bp) node {$\mu$};
\draw [decorate,postaction={decorate,draw,red,decoration={markings,mark=at position 0.5 with {\arrow{>}}}}] (v1) ..controls (165.94bp,196.07bp) and (175.13bp,201.11bp) .. (v3);
\draw [decorate,decoration={coil,aspect=0.3,segment length=1mm}] (v3) ..controls (242.34bp,247.65bp) and (276.71bp,275.25bp) .. (l4);
\draw [decorate,postaction={decorate,draw,red,decoration={markings,mark=at position 0.5 with {\arrow{>}}}}] (v3) ..controls (204.08bp,187.33bp) and (203.92bp,172.56bp) .. (v2);
\draw [decorate,decoration={coil,aspect=0.3,segment length=1mm}] (v4) ..controls (284.58bp,199.89bp) and (293.69bp,210.1bp) .. (l5);
\draw [decorate,decoration={coil,aspect=0.3,segment length=1mm}] (v4) ..controls (282.59bp,143.96bp) and (295.76bp,122.04bp) .. (l6);
\draw [decorate,decoration={coil,aspect=0.3,segment length=1mm}] (v4) ..controls (236.65bp,161.26bp) and (230.36bp,157.78bp) .. (v2);
\draw [decorate,decoration={coil,aspect=0.3,segment length=1mm}] (v4) ..controls (237.63bp,193.99bp) and (230.06bp,199.27bp) .. (v3);
%
\end{tikzpicture}
[7]:
ffd = copy.deepcopy(fd).add_rule("diagram { layout : dot ; direction : up}")
DotRender(ffd).render(resolution=100,show=False)
[7]:
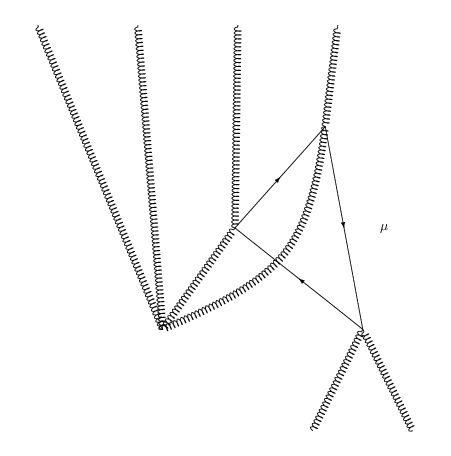
[8]:
ffd = copy.deepcopy(fd).with_style("layout : dot ; direction : right")
DotRender(ffd).render(resolution=100,show=False)
[8]:
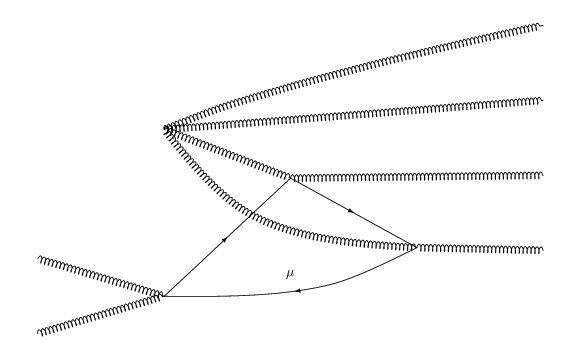
[ ]: